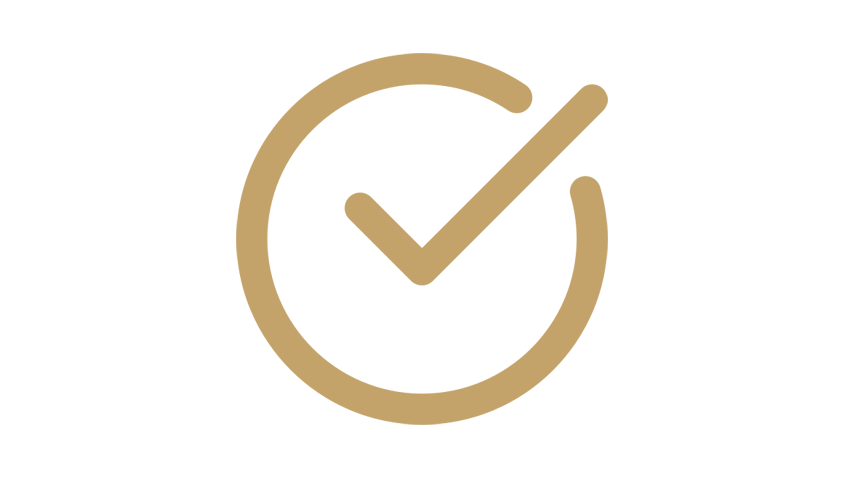
Tried and Tested
Used in over 100,000 systems worldwide.
Number Plate Recognition Engine with Software Development Kit
Used in over 100,000 systems worldwide.
Worldwide recognition of vehicle plates, make, model, and color with a single integration.
Four updates released per year.
From small through medium, to large and complex systems.
SDK supports either data input.
Runs on Windows and Linux.
#include "gxsdldr.c"
#include "cmanpr.h"
// If an error occurred it displays the error and terminates the program
void term_if_err(int st) {
int err_code;
wchar_t err_str[256];
if(st) return; // No error occurred
// Displays error code and description
err_str[(sizeof(err_str)/sizeof(wchar_t))-1] = 0;
gx_geterror(&err_code, err_str, (sizeof(err_str)/sizeof(wchar_t))-1);
fprintf(stderr, "GX error (%x) occurred: %ls\n", err_code, err_str);
// Terminates the program
exit(1);
}
int main(void) {
struct gxHANDLE anprhandle, imagehandle;
struct gxIMAGE *image;
struct cmNP *anprresult;
int st;
// Opens the ANPR module with EUR region
st = gx_openmodule(&anprhandle, L"cmanpr", L"eur");
term_if_err(st);
// Opens the image module, allocates the image structure and load the image
st = gx_openmodule(&imagehandle, L"gximage", L"default");
term_if_err(st);
st = gx_allocimage(imagehandle, &image);
term_if_err(st);
st = gx_loadimage(imagehandle, image, L"image.jpg", GX_UNDEF);
term_if_err(st);
// Finds the first license plate
anprresult = 0;
st = cm_findfirst(anprhandle, image, &anprresult);
term_if_err(st);
if(anprresult) {
char countryCode[256]="";
// Get short country code
st = cm_getcountrycode(anprhandle,anprresult->type,(int)CCT_COUNTRY_SHORT,countryCode,sizeof(countryCode));
term_if_err(st);
// Displays the result, country code and type
printf("Plate text: %s\n", anprresult->text);
printf("Country code: %s\n", countryCode[0] ? countryCode : "No plate type");
printf("Type: %i\n", anprresult->type);
// Frees up the result
st = gx_globalfree(anprresult);
term_if_err(st);
} else {
printf("No license plate found\n");
}
// Frees up the resources
st = gx_unrefimage(imagehandle, image);
term_if_err(st);
st = gx_unrefhandle(&imagehandle);
term_if_err(st);
st = gx_unrefhandle(&anprhandle);
term_if_err(st);
return 0;
}
#include "gxsdldr.cpp"
#include "cmanpr.h"
int main() {
try {
// Creates the ANPR object with EUR region
cm::cmAnpr anpr("eur");
// Creates the image object and load the image
gxImage image;
image.Load("image.jpg");
// Finds the first license plate
if(anpr.FindFirst(image)) {
// Get short country code
gxOutStr countryCode = anpr.GetCountryCode(anpr.GetType(),(int)cm::CCT_COUNTRY_SHORT);
std::wstring wCountryCode = countryCode.empty() ? L"No plate type" : countryCode;
// Displays the result, country code and type
std::wcout << L"Plate text: " << anpr.GetText() << std::endl;
std::wcout << L"Country code: " << wCountryCode << std::endl;
std::wcout << L"Type: " << anpr.GetType() << std::endl;
} else {
std::wcout << L"No license plate found" << std::endl;
}
} catch(gxError &e) {
std::wcerr << L"GX error (" << e.GetErrorCode() << ") occurred: " << e.GetErrorString() << std::endl;
return 1;
}
return 0;
}
using System;
using gx;
using cm;
namespace carmen
{
class MainClass
{
public static void Main(string[] args)
{
try {
// Creates the ANPR object with EUR region
cmAnpr anpr = new cmAnpr("eur");
// Creates the image object and load the image
gxImage image = new gxImage();
image.Load("image.jpg");
// Finds the first license plate
if (anpr.FindFirst(image)) {
// Get short country code
String countryCode = anpr.GetCountryCode(anpr.GetType(), (int)CC_TYPE.CCT_COUNTRY_SHORT);
countryCode = countryCode.Length > 0 ? countryCode : "No plate type";
//Displays the result, country code and type
Console.WriteLine("Plate text: {0}", anpr.GetText());
Console.WriteLine("Country code: {0}", countryCode);
Console.WriteLine("Type: {0}", anpr.GetType());
} else {
Console.WriteLine("No license plate found");
}
} catch(gxException) {
Console.Error.WriteLine("GX error (" + gxSystem.GetErrorCode() + ") occured: " + gxSystem.GetErrorString());
Environment.Exit(1);
}
}
}
}
import com.adaptiverecognition.gx.*;
import com.adaptiverecognition.cm.*;
public class cmanpr {
static {
try {
System.loadLibrary("jgx");
System.loadLibrary("jcmanpr");
} catch(UnsatisfiedLinkError e) {
System.err.println("Native code library failed to load." + e);
System.exit(1);
}
}
public static void main(String argv[]) {
try {
// Creates the ANPR object with EUR region
cmAnpr anpr = new cmAnpr("eur");
// Creates the image object and load the image
gxImage image = new gxImage();
image.Load("image.jpg");
// Finds the first license plate
if(anpr.FindFirst(image)) {
// Get short country code
String countryCode = anpr.GetCountryCode(anpr.GetType(),jcmanprConstants.CCT_COUNTRY_SHORT);
countryCode = countryCode.length()>0 ? countryCode : "No plate type";
// Displays the result, country code and type
System.out.println("Plate text: " + anpr.GetText());
System.out.println("Country code: " + countryCode);
System.out.println("Type: " + anpr.GetType());
} else {
System.out.println("No license plate found");
}
// Frees up resources
anpr.delete();
image.delete();
} catch(RuntimeException e) {
System.err.println("GX error (" + String.format("0x%08x",gxSystem.GetErrorCode()) + ") occured: " + gxSystem.GetErrorString());
System.exit(1);
}
}
}
Imports System
Imports gx
Imports cm
Module Main
Sub Main()
Try
' Creates the ANPR object with EUR region
Dim anpr As cmAnpr = New cmAnpr("eur")
' Creates the image object and load the image
Dim image As gxImage = New gxImage("default")
image.Load("image.jpg")
' Finds the first license plate
If anpr.FindFirst(image) Then
' Get short country code
Dim countryCode As String
countryCode = anpr.GetCountryCode(anpr.GetType(), CC_TYPE.CCT_COUNTRY_SHORT)
If countryCode.Length = 0 Then countryCode = "No plate type"
' Displays the result, country code and type
Console.WriteLine("Plate text: {0}", anpr.GetText())
Console.WriteLine("Country code: {0}", countryCode)
Console.WriteLine("Type: {0}", anpr.GetType())
Else
Console.WriteLine("No license plate found")
End If
Catch ex As gxException
Console.Error.WriteLine("GX error (" + gxSystem.GetErrorCode().ToString() + ") occured: " + gxSystem.GetErrorString())
Environment.Exit(1)
End Try
End Sub
End Module
This type of license is recommended for access control projects with less than 300 parking places.
Via this option, you can adapt your licensing to the processing capacity of your hardware (see table below).
Single License (Image/Sec) | Dual License (Image/Sec) | Quad License (Image/Sec) | |
---|---|---|---|
i3 processor
|
1 - 5 | 2 - 10 | 4 - 20 |
i5 processor
|
3 - 7 | 6 - 14 | 12 - 28 |
i7 processor
|
5 - 10 | 10 - 20 | 20 - 40 |
Ez a form a termékekhez. Minden terméknél a preferred product type-ot fix értékkel egy hidden mezőben el kell helyezni. Az Aktív form nevek kezdődjenek így: AR_PRODUCT_(product név)
Our sales & product experts are here to help you. Contact us or find an affiliate near your location.
View our representatives on a mapBy being Kazakhstan’s national road management operator, KazAvtoZhol faces a great challenge: automating the tolling of over 10,000 km of roads as part of the “Western Europe – Western China” framework project. The first signs are already visible on three Kazakh highways, now offering automated tolling and 20 weigh-in-motion stations thanks to Adaptive Recognition ANPR cameras and software.
Ugandan Immigration officers can now effectively control entry into the country with Adaptive Recognition’s Osmond passport reader and ID scanner devices.
Our Carmen® Freeflow software is the backbone of the traffic monitoring system at the Interstate 77 (I-77) highway in the United States for drivers and operators alike.
Traffic management in the City of Baguio in the Philippines is handled by Adaptive Recognition’s state-of-the-art ANPR software, Carmen® Freeflow.
The streets of the Mexican city of Puebla are now safer thanks to Adaptive Recognition’s mobile MicroCAM M402 ANPR camera units.
The acronym REPUVE synthesizes the name of a public institution: the Public Vehicle Registry. This state agency controls the circulation of vehicles on the streets and highways throughout the Mexican territory. Its principal community contribution is to help the police authorities to combat the illegal theft of vehicles and to return the seized units to their owners.
Adaptive Recognition’s contribution to the REPUVE initiative: M402 MicroCAM mobile ANPR camera units.
Thanks to the cooperation between Siett Cartago SAS and Deviteck, vehicle speed is monitored and enforced by Adaptive Recognition SmartCAM ANPR cameras.
Access control to this public university is aided by the M202 models of Adaptive Recognition’s MicroCAM mobile ANPR cameras.
A member of the Radisson Hotels chain (formerly Capital Plaza Hotel) in Port of Spain, Trinidad and Tobago, is using Adaptive Recognition Combo Smart passport readers and ID scanners to accelerate guest registration processes.
With the cooperation between Systems Enterprise and Adaptive Recognition, Combo Smart passport readers and ID scanners guard several Nicaraguan border crossing points.
The Malaysian company of Entrypass Corporation Sdn Bhd now has a visitor management system comprising Adaptive Recognition Combo Smart ID readers.
Client registration at this Australian boat club is now faster and more convenient thanks to Adaptive Recognition Combo Scan ID reader units.
Thanks to Hajonsoft, Shamal Travels in Mauritius can now perform fast and advanced ID registry and verification with Adaptive Recognition’s Combo Smart passport readers.
Travelers choosing South African Malek Travel & Tours now enjoy a better ID registration and verification experience thanks to Adaptive Recognition’s Combo Smart passport readers.
Being owned by the first Spanish tourism company, Globalia, and mainly being present in Spanish-speaking regions such as the Iberian Peninsula, the Caribbeans, and Northwestern Africa, Be Live Hotels is a network of four- and five-star hotels offering unique experiences for their guests. To better the guest registration process, the hotel chain’s Caribbean branch first purchased two Combo Smart advanced passport readers and ID scanners from Adaptive Recognition, soon to be followed by more purchases.
Established in 1999, SSK Technologies provides and develops security and surveillance solutions for all prime sectors, including but not limited to government, hospitality, construction, telecom, education, and healthcare. For the Expo Dubai 2020 event, the company created a unique, unified platform called VDS to manage UVSS, ACS, VMS, etc., security systems simultaneously and handle ANPR information acquired from Adaptive Recognition’s state-of-the-art ANPR/LPR cameras.
Founded in 2009, New Zealand-based Focus Digital Security Solutions is an experienced system integrator in the Oceania region in designing and deploying advanced CCTV, access control, and license plate recognition solutions. By implementing Adaptive Recognition’s industry-leading ANPR software, Carmen® Freeflow, with Digifort’s advanced Video Management System and being in close cooperation with Auror, a retail crime intelligence and loss prevention platform, Focus Digital pioneered a complex video surveillance system preventing major fuel station chains from falling victim to drive-off related fuel theft.
Founded in 2013, 6SS is a developer and provider of security solutions, helping businesses with video analytics, intelligent platforms, plug-ins, and third-party integrations worldwide. With their solution, a prominent Egyptian client integrated 15 regular IP cameras into an advanced system comprising a straightforward data analytics platform and Adaptive Recognition’s industry-leading license plate recognition solution, Carmen® Freeflow.
Thanks to upgrading to the latest, most advanced technology in vehicle access control, parking at the College of the North Atlantic Qatar is now managed by the tandem of Adaptive Recognition’s Vidar high-speed traffic monitoring cameras and Carmen® Freeflow.
Access control of the headquarters building of Qatar Gas is now managed by Adaptive Recognition’s Vidar HDx traffic monitoring camera models and industry-renowned Carmen® Freeflow.
ZOFRI is a 240 ha duty–free zone in the Chilean city of Iquique. Housing more than 1,600 operating companies, this special zone is safeguarded by a complex business intelligence system explicitly developed for ZOFRI by BTCO S.A. Integrated into the system is an access control subsystem that has been upgraded with 30 Adaptive Recognition SmartCAM ANPR/ALPR cameras in 2020 to reduce time spent at the barriers and filter out unauthorized vehicles from entering the premises.
Our ID readers help the work of customs officers in Georgia.
ID readers for client registration have been installed at the Casino Hotel Medea in Batumi, Georgia, member of the Pasha Global network of casinos.
For the United Nations Development Programme, ANPR/ALPR systems have been installed at border crossing points in Georgia.
ID readers for border crossing points at international airports and radar systems for traffic control have been installed in Georgia.
With the aid of 120 Adaptive Recognition ParkIT ANPR/ALPR cameras and world-renowned Carmen® ANPR/ALPR software, Apriclass-GELB, under the supervision of Pablo Maggiore, Product Manager of Apriclass, managed to upgrade the access control systems of many major Argentinian airports across the country. Now passengers can enjoy a faster, smoother, more reliable parking experience.
With the help of the Technical United Co., the Saudi Customs offices managed to upgrade the border control at the borders of Saudi Arabia with sophisticated X-ray scanner systems. Our FreeWAY ANPR cameras read and recognize license plates to be compared with the Saudi Arabian vehicle database alongside the X-ray car scanners. A more complex X-ray system with FreeWAY, SmartCAM, and ContainerCAM traffic monitoring cameras was also implemented to read the trucks’ license plates and container codes. A simpler version of this system – consisting of X-ray scanners, SmartCAMS, and ContainerCAMs – was installed on gantries at specific Saudi Arabian border crossing points.
“The UVIScan system, combined with Adaptive Recognition‘s additional ANPR components, proved to be excellent in replacing the traditional way of under vehicle inspection. We were so satisfied that we decided to drop renting the solution and opt for permanent fixtures. With the help of SecureOne, we installed two fixed UVIScan (Under Vehicle Inspection) systems plus ANPR provided by Adaptive Recognition in 2013, which have been working perfectly ever since.“
– Freddy Bosman, Head of Security Systems at the European Council Headquarters, 2015, Brussels, Belgium
By upgrading to a new access control system comprising of Carmen® Freeflow and a set of FreewayCAM ANPR cameras, the Curtis Garden Gate of the UK Houses of Parliament can now ensure a fast, safe, and fully automated admission of representatives and other parliament employees to designated parking lots.
At the Riyadh head offices of the Directorate of General Intelligence of Saudi Arabia, a total of 12 entry and exit gates are safeguarded by Adaptive Recognition ParkIT traffic monitoring cameras integrated into KASHEF, the Saudi central vehicle database.
The parking of the Governor’s house at the Emirate of Madinah Province has been upgraded with our ParkIT ANPR parking solution.
Teaming up with 4Axis, Captaurus successfully installed our ParkIT access control cameras to the parking areas of the CMC Tower, the headquarters of Cayan Group.
The Office of HH Crown Prince Mohammed Bin Salman at the aviation site of the Saudi Arabian Ministry of Defense is protected by Adaptive Recognition’s tried and tested ParkIT ANPR cameras.
Located within the Riyadh campus of the King Saud University, the Riyadh Techno Valley is often nicknamed the Silicon Valley of Saudi Arabia to transform the country into a knowledge-based economy. To fit this purpose, the building complex adapted our ParkIT smart ANPR cameras for its parking facilities.
Commissioned by the Bin-Dayel Group, Captaurus installed a complex parking system for the international parking lot of the King Khalid Airport of Riyadh, consisting of Adaptive Recognition’s highly reliable ParkIT ANPR cameras.
Our Carmen® software provides vehicle recognition for access control at European Central Bank facilities.
By upgrading to a pay-by-plate solution using our SmartCAM ANPR cameras, the ferry service operated by the Læsø Municipality is now running more cost-efficiently thanks to faster turnover rates and the better dispersion of vehicles on board the ships.
The Royal Commission of Al-‘Ula developed a centralized command and control center with a 911 emergency response operation. The parking areas and entrances to the center’s campus and buildings use 15 of our ANPR cameras licensed under the Tyco brand connected to a server-based ANPR solution.
Teaming up with Abaja Contracting Establishment, 6SS successfully upgraded the Aramco Jizan Bulk Plant access control system with the HDx Digital model of our FreeWAY ANPR camera.
Located in Al Khor, Qatar, and opened in February 2020, the Al Bays Stadium housed the 2022 FIFA World Cup opening ceremony and several football matches. Over 2,800 ANPR cameras – including ours – safeguard the entering and exiting of the audience’s vehicles in the stadium’s parking facilities, all of which use the Milestone XProtect Expert virtual machine system in tandem with the ANPR system of 6SS.
The parking garage comprising 270 spaces and is located under this shopping mall complex, uses the access control system comprising of our Carmen® ANPR software and three FreeWAY ANPR cameras.
ADCB, one of the most relevant commercial banking companies in the United Arab Emirates, had its access control system to its parking premises installed with four of our FreeWAY ANPR cameras and got them integrated into our Carmen® ANPR software.
Being a main distributor of specialized building materials for many clients, Teejan General Trading LLC considers safe warehousing of utmost importance. As such, the company has decided to safeguard the entrances to its strategically located warehouse in Dubai with two of our FreeWAY cameras and hook them up to our industry-leading ANPR software, Carmen®.
The parking lot for the Dubai offices of this retail center development company uses two of our products: a FreeWAY camera and Carmen® Freeflow ANPR software.
Opened in 2018, the First Avenue Mall & Hotel is an environmentally friendly, contemporary, European-style mall stretched over 46,000 square meters. Its underground parking garage is equipped with our solution, consisting of an Adaptive Recognition FreeWAY traffic monitoring camera and the Carmen® Freeflow ANPR software.
Elmacs Co. LLC is a significant player in the United Arab Emirates in installing and maintaining mechanical, electrical, plumbing, and firefighting systems. Access to parking spaces at the company’s offices in Dubai is now safeguarded by four FreeWAY cameras linked to our industry-leading ANPR software, Carmen®.
At the main GECO complex, located in Sharjah, the United Arab Emirates, the access control system is managed by Carmen® Freeflow linked to our two FreeWAY ANPR cameras.
The facility’s access control system features our ParkIT ANPR cameras.
The shopping mall’s parking access control system features our ParkIT ANPR cameras.
Axiome has installed several ParkIT license plate cameras with our Carmen® ANPR software for its automated parking management system. In Canada, these systems operate at Hilton Pearson, SOHO Hotel and at the Quebec Airport, for example.
Advanced Detection Technology has been employing our Carmen® ALPR / ANPR engine as a key module for its comprehensive access control solutions for over a year and has experienced great results and positive customer feedback. With this success, Advanced Detection Technology has decided to integrate the ALPR technology as a built-in, value-added feature to its LowCam™ Under Vehicle Inspection Systems.
„Overall, the use of the Carmen® engine has produced a very manageable, predictable, cost effective ALPR solution which we utilize as a valuable, technology based efficiency component, of the services we offer our customers. Our company has leveraged the Carmen® engine for more than 5 years and during that period of time worked extensively with Adaptive Recognition to obtain the highest amount of technology and business value while working together through several technical challenges. Adaptive Recognition has been a supportive and responsive partner and we receive value beyond the obvious technical value stated above. Working with a vendor partner that is truly invested in the successful deployment of technology creates additional value for us.”
In US Marine Container terminals, our Carmen® ANPR software identifies license plates passing through the smart-Tecs OCR portals.
„We have been successfully implementing this solution with our own Gate Operating Software (GOS). We’ve experienced over 97 percent successful recognition rate of license plates. We are extremely pleased with the results and are completely satisfied with the efficiency of the Carmen ANPR software.” – Lawrence Chan, Vice President of Operations, smart-Tecs, LLC
„Dating back to 2015, Kapsch TrafficCom USA has partnered with Adaptive Recognition America (ARA) to provide the Carmen® FreeFlow OCR Engine as part of our product offerings in North America. We have deployed the Carmen® FreeFlow package at multiple sites and for several customers with consistently strong results.
ARA has worked closely with our development team to provide licensing key configurations that would allow Kapsch to deploy multiple Carmen® instances to support high volume AET sites and still be able to meet accuracy and delivery time KPIs.” – Thomas Kramek, Senior Program Director, Kapsch TrafficCom USA Inc.
JAI, Inc. (USA) has successfully utilized the Carmen® ANPR software in numerous ITS and tolling systems & operations for the identification, recognition, and reading of vehicle license plates.
CARMEN® engine has been used to develop high-performance back-office systems that routinely process 100,000 + transactions or more daily. These high-volume systems must perform consistently on a 24-hour, 365-day basis under all prevailing weather and ambient conditions throughout the year. These systems operate with an exemplary error rate of less than 1% (!).
„This Adaptive Recognition product is one of the high-performance components to our “State of the art” revenue collection and violation enforcement systems in wide deployment globally.” – Frank Long, Director at JAI Traffic Solutions
Our professional number plate recognition software, Carmen® is integrated into Axxonsoft’s solution, used in the Humriya Free Zone (United Arab Emirates).
Carmen®, our professional license plate recognition software, integrated into Axxonsoft’s solution is used at the Sharjah Airport Free Zone, in the United Arab Emirates.
At the Serengeti Golf Estate in South Africa, our license plate recognition solution is used, integrated into Axxonsoft’s system.
In Malaysia’s Penang safe city project, license plate recognition is performed by Axxonsoft’s solution, using the Carmen® Freeflow ANPR software.
“We have been using your products for quite some time and may have been one of your first
customers in the US to leverage the Carmen ACCR product. Since then we have added usage to
include semi-truck Chassis IDs and have also integrated the license plate OCR solution. Recently,
we have started to work with the DOT OCR product and are getting very good results at our test
site!
I believe that our solutions offering will grow and a large part of that growth is due to our
inclusion of your products. Thank you again for all that you do and keep up the great work!”
– Mark Anderson, Business Director, August 25, 2020, USA
CatchSystem installed our ANPR and make & model recognition technology on the A12 highway in the Netherlands. In the bus lane, automated vehicle identification is used for enforcement purposes.
Adaptive Recognition’s Freeway HDx 2nd generation ANPR cameras manage vehicle recognition at the Dubai Marina Mall.
Built and managed by Ascendi, the traditional toll gates installed on the Portuguese highway of A13 and A13-1 between Tomar and Coimbra/Condeixa use our flagship Carmen® Freeflow ANPR software for lightning-fast and accurate license plate recognition.
Because speed is critical in any toll payment system – to minimize bottlenecks at toll points and to comply with the strict contract requirement related to transaction times – N6 Concessions Ltd is using FreewayCAM ANPR cameras and Carmen® Freeflow ANPR software to extend the tolling system with number plate recognition capabilities, this way ensuring the required level of service.
Our license plate recognition technology is used in the automated tolling systems of A1 and A226 highways.
BTCO S.A. is a system integrator company with projects delivered to end-users in Chile, Argentina, Uruguay, Peru, and Colombia. In 2020, BTCO acquired 30 Adaptive Recognition SmartCAM ANPR/ALPR cameras to upgrade the access control subsystem of a complex business intelligence system developed for ZOFRI, a 240-ha duty–free zone in the Chilean city of Iquique that houses more than 1,600 operating companies. Since then, time spent at the barriers has been significantly reduced, and unauthorized vehicles are easily prevented from entering the premises.
Being the ambassador of projects creating the world of tomorrow, IBI Group upgraded the Guadalajara–Colima highway tolling system in Mexico with Adaptive Recognition’s ANPR cameras and software to provide a state-of-the-art electronic tolling system. With IBI Group’s solution, the highway operator can also continuously monitor entry and exit times at the toll plazas to deliver the KPIs required by the federal government.
Kineo – Los Portales Estacionamientos parking solutions use Adaptive Recognition’s ParkIT system with FreewayCAM cameras and Carmen® ANPR software.
PYV Technology in Mexico uses our Carmen® Freeflow ANPR software in access control applications.
Himmelsbach uses our automatic container code recognition and railway code identification software in various projects.
This casino in Liechtenstein uses a smart ID scanner to collect guest data automatically – complying with the regulations and speeding up the check-in process.
This train station in Switzerland uses a digital parking system for their travelers. Incoming vehicles are identified by their license plates, using ANPR technology.
This casino in Switzerland uses a digital parking system for enhanced convenience and security. Incoming vehicles are identified by their license plates, using ANPR technology.
At the borders of Kosovo, Combo Smart passport readers are used for document inspection and FreewayCAM ANPR cameras.
This shopping mall in Baku uses Adaptive Recognition ANPR technology in its vehicle parking access control system.
Ventech Systems Gmbh (now part of GoodYear) is a developer of professional vehice inspection systems. In some of their projects they use our license plate recognition technology for automatically identifying vehicles.
Ventech Systems Gmbh (now part of GoodYear) is a developer of professional vehice inspection systems. In some of their projects they use our license plate recognition technology for automatically identifying vehicles.
Ventech Systems Gmbh (now part of GoodYear) is a developer of professional vehice inspection systems. In some of their projects they use our license plate recognition technology for automatically identifying vehicles.
Ventech Systems Gmbh (now part of GoodYear) is a developer of professional vehice inspection systems. In some of their projects they use our license plate recognition technology for automatically identifying vehicles.
Ventech Systems Gmbh (now part of GoodYear) is a developer of professional vehice inspection systems. In some of their projects they use our license plate recognition technology for automatically identifying vehicles.
Ventech Systems Gmbh (now part of GoodYear) is a developer of professional vehice inspection systems. In some of their projects they use our license plate recognition technology for automatically identifying vehicles.
Ventech Systems Gmbh (now part of GoodYear) is a developer of professional vehice inspection systems. In some of their projects they use our license plate recognition technology for automatically identifying vehicles.
Ferrovial is an expert in highway security. The company integrated our Carmen® Freeflow ANPR engine and installed it in various traffic systems in Portugal (A28 Norte Litoral, A23 Via do Infante) and the USA (NTE TEXpress Lanes, NTE 35W, LBJ TEXpress Lanes, I-77 Express)
In mission-critical environments, security is a must. In this project, an under-vehicle inspection system helps the security staff to create protection against explosives, weapons, narcotics, and other contraband products hidden under vehicles. Our license plate recognition technology identifies each vehicle.
On Greek highways, a modern system is in operation to enforce unauthorized usage of emergency lanes. The system includes our TrafficSpot data gathering solution, EnforceCAM and SmartCAM ANPR cameras, and our traffic database middleware, GDS.
At the Dutch Police, police vehicles are equipped with mobile ANPR systems that are capable of recognizing wanted vehicles even from a moving vehicle.
The National Police of Bulgaria use 30 S1 portable speed and traffic enforcement devices. This device is praised for its high-accuracy speed measurement sensor and its onboard processing with embedded license plate recognition and traffic enforcement modules, such as the action list function for catching wanted vehicles.
Armenia Government uses our ANPR technology to enhance general security in the country.
Parking is made easy by a ticketless solution in this shopping mall, using license plate data.
At Bayer, our ParkIT System makes sure that the entry/exit of all vehicles is quick and secure. This solution uses license plate recognition to automatically identify employees and guests.
The project is for the municipality of Tripoli in Lebanon, including a traffic light system with traffic monitoring cameras for license plate recognition and automatic detection of red light violations.
Parking and the access control of this hotel is aided by our license plate recognition system, to make it safe and convenient.
Access control is performed by professional license plate recognition at this oil company on the Azores island.
Guests of the friendly Barkhoorn campsite are ensured by the modern, ANPR-based access control system at the entry/exit points of the area.
This Danish ferry company decided to ease their passengers’ travel while they optimize their workflow and overcome some of their daily headaches – all at once. Products used: Intellio Initio Dome CCTV cameras, and Combo Scan ID scanners.
In the Stena Line shipping port (Karlskrona, Sweden), there is a lot of goods coming through each day. A new project, called rePORT is being implemented to automate processes and make workflow smarter – in Stena Line’s vision there is a smart port system where freights are properly tracked and personnel’s job is aided with smart technology.
Z Energy is a New Zealand fuel distributor owning 200+ gas stations nationwide. In 2020, following a successful Pay-by-Plate pilot, formerly branded Fastlane, the company opted for a pay-by-plate solution by Focus Digital Security Solutions for 62 of its sites, comprising CCTV cameras and video management systems with Carmen® Freeflow ANPR software.
What makes a parking system smart? This well-known hotel in Copenhagen is well aware of it – and we show the details in this case study.
Our ultra-compact Combo Scan ID scanners are used in Korea to register customers’ travel document data to complete duty-free purchases.
At Budapest Airport, our ID & passport verification technology is used both in regular border control and also in Automated Border Control e-gates to perform quick and hassle-free border crossing while taking security to a next level.
After we delivered a fleet of portable S1 speed cameras with onboard ANPR, Tunisian forces captured 150 vehicles in only 1 month.
Protection for sporting and cultural events attracting masses have always been a security challenge – but not with IntelliSport.
Protection for sporting and cultural events attracting masses have always been a security challenge – but not with IntelliSport.
Adaptive Recognition ANPR technology serves as the backbone of the Hungarian nationwide road safety and traffic control system.
Adaptive Recognition provides ANPR technology at the Tunel de Oriente in Colombia.
Miskolc is a major county capital in Hungary, on 250+ km2 with more than 150,000 citizens. Organizing centralized security in such a large area is definitely a challenge, but not with smart technology.
A route with a daily traffic of almost 20,000 vehicles (!) have a crucial need: the smooth flow of traffic with no traffic jams, all these in a way that remains safe. Learn how.
According to the EU’s directive, all external borders of the EU will become biometric by 2022. As such, the Hungarian Police opted for Combo Smart Kiosk devices integrated into e-gates to accelerate the passport and ID document checking process while ensuring that unwanted people are caught immediately.
Incoming vehicles are tracked; using the license plate data the system can locate lost luggages, control the entry/exit barriers, find stolen vehicles, maintain a KYC database and more.
The parking system in the world’s highest building is ensured by license plate recognition cameras and automatic, LPR-based access control.
Thailand uses mobile license plate recognition to identify vehicles anywhere on the road. MicroCAM mobile ANPR cameras, mounted on top of police cars, automatically detect and identify all vehicles based on their license plates.
ID scanners aid bank operations and avoid any losses due to unauthorized transactions. Customer data automatically populates the bank’s IT system while a forgery check is performed on personal documents.
In all branch offices, transactions and customer verification are enhanced by automatically reading and authenticating customers’ ID documents.
Our software and purpose-built cameras detect vehicles, capture images of the windscreen, and, using our AI-based proprietary software, verify the validity of the printed toll stickers on Austrian highways.
The security of money transfer is aided by ID verification.
Passport readers at the reception desk make check-in quicker and easier – this way, the hotel offers a truly advanced guest experience.
ID document scanners were installed to automatically extract data from passports, visas or ID cards. The system avoids any input errors and offers great speed compared to manual typing.
The telco company in Austria installed hundreds of Combo Smart N models to benefit from its all-in-one operation: the device automatically processes the ID documents and sends the data packages to a pre-defined server location.
Having a database of wanted vehicles, NSA can easily detect them anywhere on the road, thanks to mobile LPR. In such scenario, an LPR camera with onboard recognition scans all surrounding vehicles and automatically alerts when there is a catch.
For immigration checks and automated border control, Hong Kong’s Immigration is one of our key users. Due to the long-term cooperation, several client-specific recognition engines have been developed to comply with special local requirements.
Passport readers at the reception desk offer an excellent benefit for the hotel: guest registration reduces to a few seconds from long minutes, and the data automatically populates the hotel / PMS software.
Thanks to automated guest data entry using Adaptive Recognition passport readers, there are no more data input errors.
Our Combo Scan ID scanners are used in KYC applications at Postal Bank Slovakia.
VFS – abbreviation of Visa Facilitation Services – uses Adaptive Recognition ID/passport readers for identifying citizens and quality control of newly issued visas.
Nordea bank uses Adaptive Recognition identity verification technology in its KYC applications.
Age verification and customer registration are handled by Adaptive Recognition ID verification technology at this casino in Germany.
Zzzleepandgo offers airport hotels – modern, capsule-style rooms located at the Malpensa Airport in Italy. The access and check-in to these rooms are enhanced by ID verification.
In the South Korean Kangwon Land casino, our ID scanner devices enhance security and customer experience.
The hotel Grand Hyatt Athens uses our Combo Smart Kiosk ID scanners for quick and convenient check-in.
ScotiaBank Mexico uses our ID scanners in their KYC applications in two business units.
GRG Banking integrated our built-in passport readers in their professional self-service equipment. These kiosks are used as smart ATMs (VTMs) at the DBS Bank in Singapore.
Toll enforcement from a moving vehicle, even on remote roads with no checkpoints with fixed cameras? Using a live database connection, Hungarian authorities use MicroCAM cameras mounted on their patrol cars to identify and categorize vehicles. This innovation results from the cooperation of National Toll Payment Services PLC and Adaptive Recognition.
Adaptive Recognition Combo Smart and Osmond ID readers take care of customer data verification at the duty-free shops of Munich airport.
Our technology handles ID verification for several members of the Mercure Hotel chain.
Highways in Serbia are monitored by ANPR cameras and tolling is automatically implemented based on license plate recognition.
Casinos in Las Vegas, such as the Harrah’s deal with a lot of money – and its players have to comply with the age limit and present a valid ID. This is what our ID scanners automate during the check-in process.
Our recognition technology is used in logistics applications at Swiss Post.